Metrics
Metrics creates custom metrics asynchronously by logging metrics to standard output following Amazon CloudWatch Embedded Metric Format (EMF).
These metrics can be visualized through Amazon CloudWatch Console.
Key features¶
- Aggregate up to 100 metrics using a single CloudWatch EMF object (large JSON blob)
- Validating your metrics against common metric definitions mistakes (for example, metric unit, values, max dimensions, max metrics)
- Metrics are created asynchronously by the CloudWatch service. You do not need any custom stacks, and there is no impact to Lambda function latency
- Context manager to create a one off metric with a different dimension
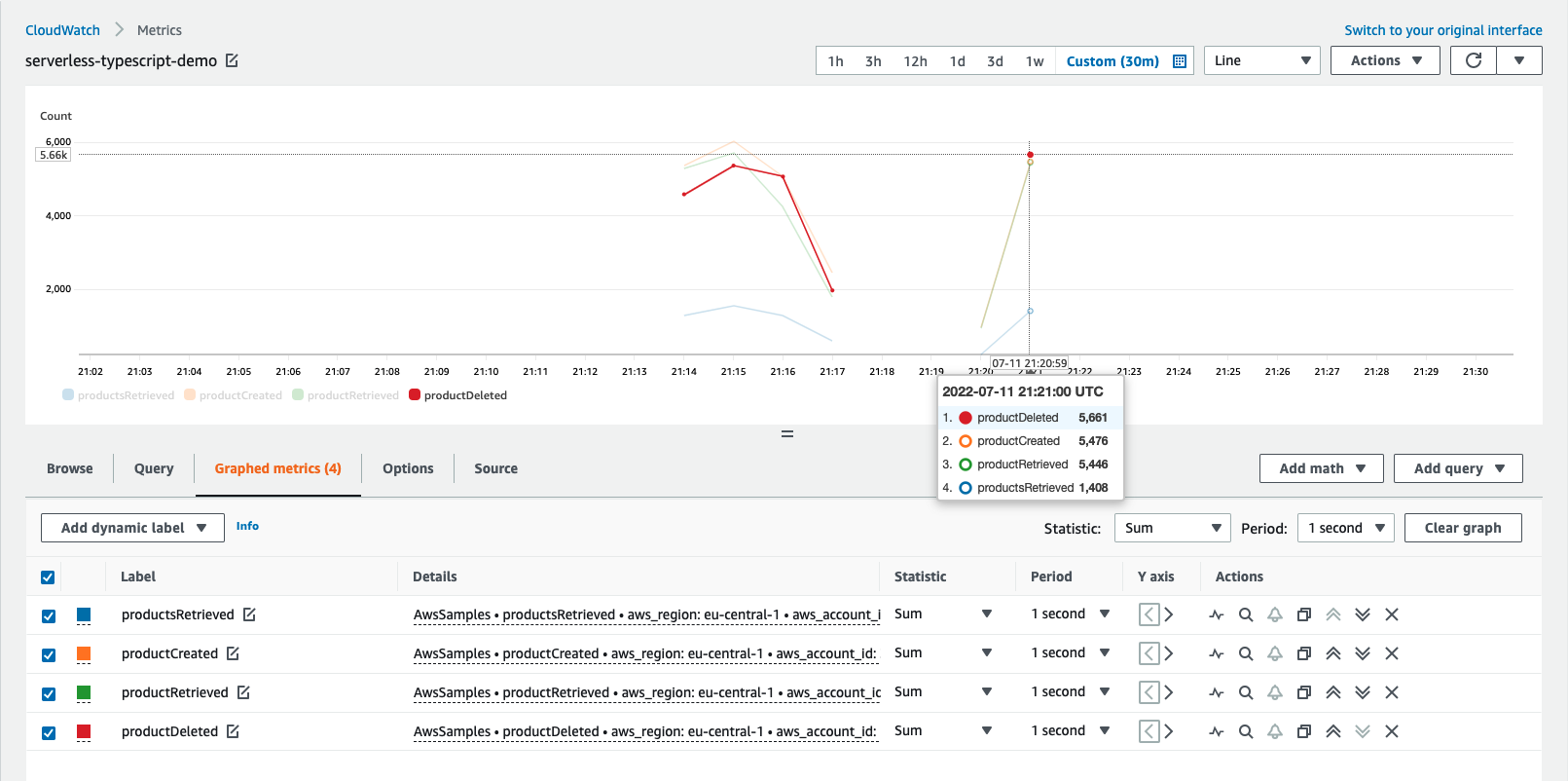
Installation¶
Powertools for AWS Lambda (.NET) are available as NuGet packages. You can install the packages from NuGet Gallery or from Visual Studio editor by searching AWS.Lambda.Powertools*
to see various utilities available.
-
AWS.Lambda.Powertools.Metrics:
dotnet nuget add AWS.Lambda.Powertools.Metrics
Terminologies¶
If you're new to Amazon CloudWatch, there are two terminologies you must be aware of before using this utility:
- Namespace. It's the highest level container that will group multiple metrics from multiple services for a given application, for example
ServerlessEcommerce
. - Dimensions. Metrics metadata in key-value format. They help you slice and dice metrics visualization, for example
ColdStart
metric by Paymentservice
. - Metric. It's the name of the metric, for example: SuccessfulBooking or UpdatedBooking.
- Unit. It's a value representing the unit of measure for the corresponding metric, for example: Count or Seconds.
- Resolution. It's a value representing the storage resolution for the corresponding metric. Metrics can be either Standard or High resolution. Read more here.
Visit the AWS documentation for a complete explanation for Amazon CloudWatch concepts.
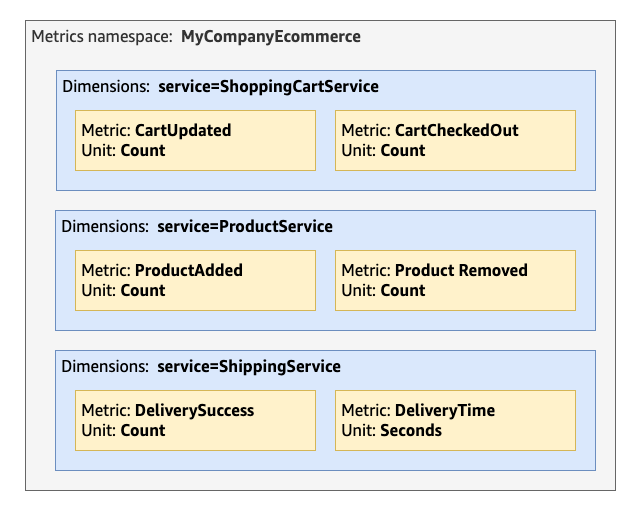
Getting started¶
Metrics
is implemented as a Singleton to keep track of your aggregate metrics in memory and make them accessible anywhere in your code. To guarantee that metrics are flushed properly the MetricsAttribute
must be added on the lambda handler.
Metrics has two global settings that will be used across all metrics emitted. Use your application or main service as the metric namespace to easily group all metrics:
Setting | Description | Environment variable | Constructor parameter |
---|---|---|---|
Service | Optionally, sets service metric dimension across all metrics e.g. payment |
POWERTOOLS_SERVICE_NAME |
Service |
Metric namespace | Logical container where all metrics will be placed e.g. MyCompanyEcommerce |
POWERTOOLS_METRICS_NAMESPACE |
Namespace |
Autocomplete Metric Units
All parameters in Metrics Attribute
are optional. Following rules apply:
- Namespace:
Empty
string by default. You can either specify it in code or environment variable. If not present before flushing metrics, aSchemaValidationException
will be thrown. - Service:
service_undefined
by default. You can either specify it in code or environment variable. - CaptureColdStart:
false
by default. - RaiseOnEmptyMetrics:
false
by default.
Example using AWS Serverless Application Model (AWS SAM)¶
1 2 3 4 5 6 7 8 9 |
|
1 2 3 4 5 6 7 8 9 |
|
Full list of environment variables¶
Environment variable | Description | Default |
---|---|---|
POWERTOOLS_SERVICE_NAME | Sets service name used for tracing namespace, metrics dimension and structured logging | "service_undefined" |
POWERTOOLS_METRICS_NAMESPACE | Sets namespace used for metrics | None |
Creating metrics¶
You can create metrics using AddMetric
, and you can create dimensions for all your aggregate metrics using AddDimension
method.
1 2 3 4 5 6 7 8 9 10 |
|
1 2 3 4 5 6 7 8 9 10 11 |
|
Autocomplete Metric Units
MetricUnit
enum facilitates finding a supported metric unit by CloudWatch.
Metrics overflow
CloudWatch EMF supports a max of 100 metrics per batch. Metrics utility will flush all metrics when adding the 100th metric. Subsequent metrics, e.g. 101th, will be aggregated into a new EMF object, for your convenience.
Metric value must be a positive number
Metric values must be a positive number otherwise an ArgumentException
will be thrown.
Do not create metrics or dimensions outside the handler
Metrics or dimensions added in the global scope will only be added during cold start. Disregard if that's the intended behavior.
Adding high-resolution metrics¶
You can create high-resolution metrics passing MetricResolution
as parameter to AddMetric
.
When is it useful?
High-resolution metrics are data with a granularity of one second and are very useful in several situations such as telemetry, time series, real-time incident management, and others.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
|
Autocomplete Metric Resolutions
Use the MetricResolution
enum to easily find a supported metric resolution by CloudWatch.
Adding default dimensions¶
You can use SetDefaultDimensions
method to persist dimensions across Lambda invocations.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
|
Flushing metrics¶
With MetricsAttribute
all your metrics are validated, serialized and flushed to standard output when lambda handler completes execution or when you had the 100th metric to memory.
During metrics validation, if no metrics are provided then a warning will be logged, but no exception will be raised.
1 2 3 4 5 6 7 8 9 10 |
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
|
Metric validation
If metrics are provided, and any of the following criteria are not met, SchemaValidationException
will be raised:
- Maximum of 9 dimensions
- Namespace is set
- Metric units must be supported by CloudWatch
We do not emit 0 as a value for ColdStart metric for cost reasons. Let us know if you'd prefer a flag to override it
Raising SchemaValidationException on empty metrics¶
If you want to ensure that at least one metric is emitted, you can pass RaiseOnEmptyMetrics
to the Metrics attribute:
1 2 3 4 5 6 7 8 |
|
Capturing cold start metric¶
You can optionally capture cold start metrics by setting CaptureColdStart
parameter to true
.
1 2 3 4 5 6 7 8 |
|
If it's a cold start invocation, this feature will:
- Create a separate EMF blob solely containing a metric named
ColdStart
- Add
function_name
andservice
dimensions
This has the advantage of keeping cold start metric separate from your application metrics, where you might have unrelated dimensions.
Advanced¶
Adding metadata¶
You can add high-cardinality data as part of your Metrics log with AddMetadata
method. This is useful when you want to search highly contextual information along with your metrics in your logs.
Info
This will not be available during metrics visualization - Use dimensions for this purpose
Info
Adding metadata with a key that is the same as an existing metric will be ignored
1 2 3 4 5 6 7 8 9 10 |
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
|
Single metric with a different dimension¶
CloudWatch EMF uses the same dimensions across all your metrics. Use PushSingleMetric
if you have a metric that should have different dimensions.
Info
Generally, this would be an edge case since you pay for unique metric. Keep the following formula in mind:
unique metric = (metric_name + dimension_name + dimension_value)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
|
Testing your code¶
Environment variables¶
Tip
Ignore this section, if:
- You are explicitly setting namespace/default dimension via
namespace
andservice
parameters - You're not instantiating
Metrics
in the global namespace
For example, Metrics(namespace="ExampleApplication", service="booking")
Make sure to set POWERTOOLS_METRICS_NAMESPACE
and POWERTOOLS_SERVICE_NAME
before running your tests to prevent failing on SchemaValidation
exception. You can set it before you run tests by adding the environment variable.
Injecting Metric Namespace before running tests | |
---|---|
1 |
|