Metrics
Metrics creates custom metrics asynchronously by logging metrics to standard output following Amazon CloudWatch Embedded Metric Format (EMF).
These metrics can be visualized through Amazon CloudWatch Console.
Key features
- Aggregate up to 100 metrics using a single CloudWatch EMF object (large JSON blob).
- Validate against common metric definitions mistakes (metric unit, values, max dimensions, max metrics, etc).
- Metrics are created asynchronously by the CloudWatch service, no custom stacks needed.
- Context manager to create a one off metric with a different dimension.
Terminologies¶
If you're new to Amazon CloudWatch, there are two terminologies you must be aware of before using this utility:
- Namespace. It's the highest level container that will group multiple metrics from multiple services for a given application, for example
ServerlessEcommerce
. - Dimensions. Metrics metadata in key-value format. They help you slice and dice metrics visualization, for example
ColdStart
metric by Paymentservice
.
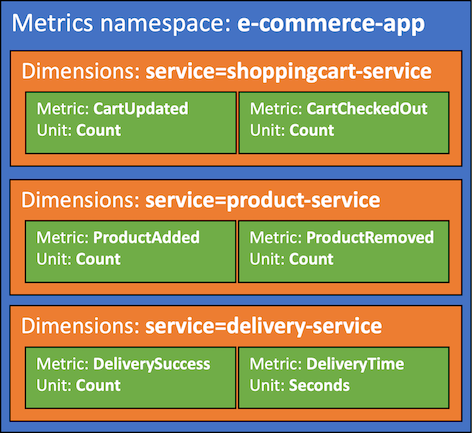
Install¶
Depending on your version of Java (either Java 1.8 or 11+), the configuration slightly changes.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
|
Getting started¶
Metric has two global settings that will be used across all metrics emitted:
Setting | Description | Environment variable | Constructor parameter |
---|---|---|---|
Metric namespace | Logical container where all metrics will be placed e.g. ServerlessAirline |
POWERTOOLS_METRICS_NAMESPACE |
namespace |
Service | Optionally, sets service metric dimension across all metrics e.g. payment |
POWERTOOLS_SERVICE_NAME |
service |
Use your application or main service as the metric namespace to easily group all metrics
1 2 3 4 5 6 7 8 9 10 |
|
1 2 3 4 5 6 7 8 9 10 11 12 |
|
You can initialize Metrics anywhere in your code as many times as you need - It'll keep track of your aggregate metrics in memory.
Creating metrics¶
You can create metrics using putMetric
, and manually create dimensions for all your aggregate metrics using putDimensions
.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
|
The Unit
enum facilitate finding a supported metric unit by CloudWatch.
Metrics overflow
CloudWatch EMF supports a max of 100 metrics. Metrics utility will flush all metrics when adding the 100th metric while subsequent metrics will be aggregated into a new EMF object, for your convenience.
Flushing metrics¶
The @Metrics
annotation validates, serializes, and flushes all your metrics. During metrics validation,
if no metrics are provided no exception will be raised. If metrics are provided, and any of the following criteria are
not met, ValidationException
exception will be raised.
Metric validation
- Maximum of 9 dimensions
If you want to ensure that at least one metric is emitted, you can pass raiseOnEmptyMetrics = true
to the @Metrics annotation:
1 2 3 4 5 6 7 8 9 10 |
|
Capturing cold start metric¶
You can capture cold start metrics automatically with @Metrics
via the captureColdStart
variable.
1 2 3 4 5 6 7 8 9 10 |
|
If it's a cold start invocation, this feature will:
- Create a separate EMF blob solely containing a metric named
ColdStart
- Add
FunctionName
andService
dimensions
This has the advantage of keeping cold start metric separate from your application metrics.
Advanced¶
Adding metadata¶
You can use putMetadata
for advanced use cases, where you want to metadata as part of the serialized metrics object.
Info
This will not be available during metrics visualization, use dimensions
for this purpose.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
|
This will be available in CloudWatch Logs to ease operations on high cardinal data.
Overriding default dimension set¶
By default, all metrics emitted via module captures Service
as one of the default dimension. This is either specified via
POWERTOOLS_SERVICE_NAME
environment variable or via service
attribute on Metrics
annotation. If you wish to override the default
Dimension, it can be done via MetricsUtils.defaultDimensions()
.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
|
Creating a metric with a different dimension¶
CloudWatch EMF uses the same dimensions across all your metrics. Use withSingleMetric
if you have a metric that should have different dimensions.
Info
Generally, this would be an edge case since you pay for unique metric. Keep the following formula in mind: unique metric = (metric_name + dimension_name + dimension_value)
1 2 3 4 5 6 7 8 9 10 11 |
|
Creating metrics with different configurations¶
Use withMetricsLogger
if you have one or more metrics that should have different configurations e.g. dimensions or namespace.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
|